Roles and Permissions in Plain English
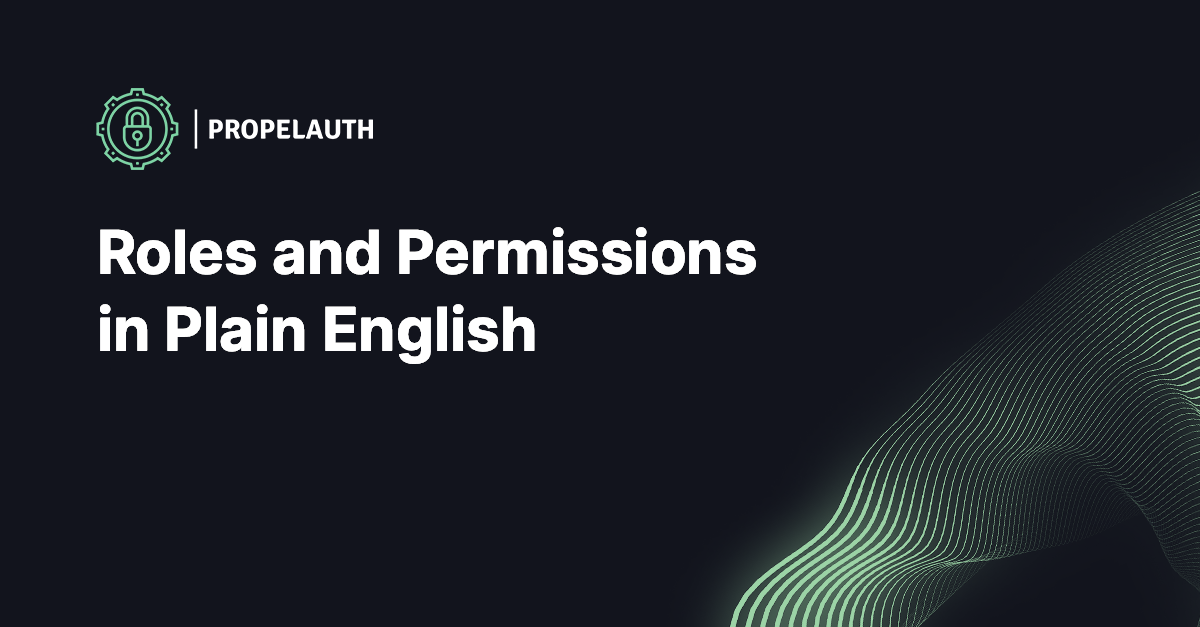
Introduction
You’re a B2B company. Let’s say you build newspaper websites for local newspapers. Your simplest product is a newspaper website where everyone can post articles and everyone can read them.
That’s not good.
Newspapers want to control who can post. There’s a just a few writers and a lot of readers. Writers can write, and readers can read. Your B2B business now has two roles, Readers and Writers.
Well, really you have three, because there’s always an owner who signed up for your B2B business and pays the bills.
It’s a newspaper, so you also want editors who can approve submissions and write editorials.
Now our newspaper B2B website has four roles: Owner, Editor, Writer, Reader. Everyone is one of those roles.
Perfect. Let’s get coding.
Coding with Roles
How do we add authorization to our application?
If a person pushes a button, we want to check if they’re authorized to push that button.
A writer posting an article might look like this piece of pseudocode:
function postArticle {
if isRole(writer) {
// post the article
}
}
An editor approving an article might look like this:
function approveArticle {
if isRole(editor) {
// approve the article
}
}
Wait, can an editor make a post too? I guess so. We should change that logic a bit:
function postArticle {
if isRole(writer) or isRole(editor) {
// post the article
}
}
What happens when we scale up? What if we have a dozen roles? Does each if statement become a giant set of ors?
function postArticle {
if isRole(writer) or isRole(editor) or isRole(manager) or isRole(intern) or isRole(guest_poster) or isRole(jims_special_role) {
// post the article
}
}
That’s a messy statement. It’s easy to forget a role, like we just did when we forgot the owner role. Another bug, and bugs should be hard to write.
How do we fix this?
Let’s make a hierarchy between the roles.
An owner is also an editor, an editor is also a writer, and a writer is also a reader.
Now these roles roll up. We can use the hierarchy to determine authorization:
function postArticle {
if isAtLeast(writer) {
// post the article
}
}
Simple.
Well, simpler. The onus is on you to create the right roles.
Permissions
With roles we were looking at who a user is. A user is a writer. A user is an editor.
What if we instead look at what a user can do? A user can post. A user can approve.
This is thinking in permissions.
A writer has permission to writePost. An editor has permissions to writePost, writeEditorial, and approvePost.
We change this:
function approveArticle {
if isRole(editor) {
// approve the article
}
}
into this:
function approveArticle {
if hasPermission(approvePost) {
// approve the article
}
}
This is easier code to write. We’re separating the act of writing code from the act of managing users.
We may not know upfront exactly which role should be able to use this feature, but we shouldn’t need to know the exact answer to that question to write this feature. With permissions we can just write code, and decide on user management later.
We already write CRUD apps, the only change to our process is to start gating each endpoint behind a unique permission. You’ll soon have a whole host of permissions like readPosts, createPosts, readDrafts, approveDraft, etc.
With permissions you have a separation of concerns, a programmer-term that programmers love. (It’s true, ask them.)
Roles and Permissions
Now we tie them together:
- Give roles to users.
- Give permissions to code.
- Tie roles to permissions in the PropelAuth dashboard.
We have an editor role, which has a host of permissions. Every user who is given the editor role automatically has those permissions.
The best part is we can very easily create new roles from customer requests. Do they want an assistant editor who can approve posts, but can’t write editorials? No problem. Make a role called AssistantEditor that only has one permission: approvePost. Need an auditor? Make a role that has all the read* permissions you can find.
We can add whole new roles—whole new pieces of functionality—to your B2B business without a single line of code. Just a few minutes in the PropelAuth dashboard.
That’s nice.
I only get one exclamation point per post, and I’m going to use it here.
That’s nice!
But you may have some questions
But you may have some questions.
Why have roles and permissions and not just roles?
Sometimes roles have a straightforward mapping from role to the activities they can do. An editor has permission to edit. A writer has permission to write. You might not think you need permissions when things are this clear.
But sometimes the mapping isn’t clear. An intern can…intern? A junior developer can read/write the test database and open PRs on Github. A senior developer can approve PRs and read the production database.
As you build out your application beyond the “hello world” stage, you’ll find more and more that permissions make your app easier to scale.
Why have permissions and roles and not just permissions?
Can we just skip roles and just assign permissions directly to users?
Sure. But it gets time-consuming fast.
Say a new database appears that needs to be accessed by all developers? With roles, we can just add an accessAnalyticsDB permission to the developers role. Now all those programmers automatically have access to what they need. We don’t have to manually add that permission to a dozen/hundred/thousand programmers. We’re gonna miss a few if we try that.
A little work upfront makes for an easier life down the road.
Roles and Permissions are powerful
I was a contractor at Bank of America. The bottom of the heap. I had three roles and a dozen permissions that came with them. My boss didn’t think about permissions, just roles. He assigned me roles and trusted that the roles → permissions were set up correctly under the hood.
It was also easy to diagnose. If I tried to access a code server and couldn’t, my boss only had to look at my roles and permissions, and quickly compare them to his other employees who had access. Did he miss a role? It was easy to add. He could fix it with no code, no tickets to sysadmins, and no manually adding me to some server’s .ssh setup. It was all easy to self-diagnose.
Imagine all your clients managing their own users and their own problems. Imagine all those clients not bugging you.
If they do need to contact you, imagine being able to diagnose those authorization problems through a simple, visual dashboard on PropelAuth. No need to trawl through code. Heck, you don’t even have to be a programmer.
In sum
Give roles to people.
Give permissions to code.
Match roles to permission in our super-easy dashboard.
Let PropelAuth solve your complex B2B authentication and authorization problems. You can focus on programming your business logic. We’ll take care of the boring parts¹.
¹ - The boring parts include but are not limited to Two Factor Authentication, MFA, SAML, Passwordless logins with magic links, SSO/Social Login, B2B organization management, Slack integration, a testing environment, libraries for all your favorite frontend and backend languages and frameworks, a beautiful and customizable UI, and a React component library for total customization (last one coming soon).