PropelAuth Now Supports Next.js
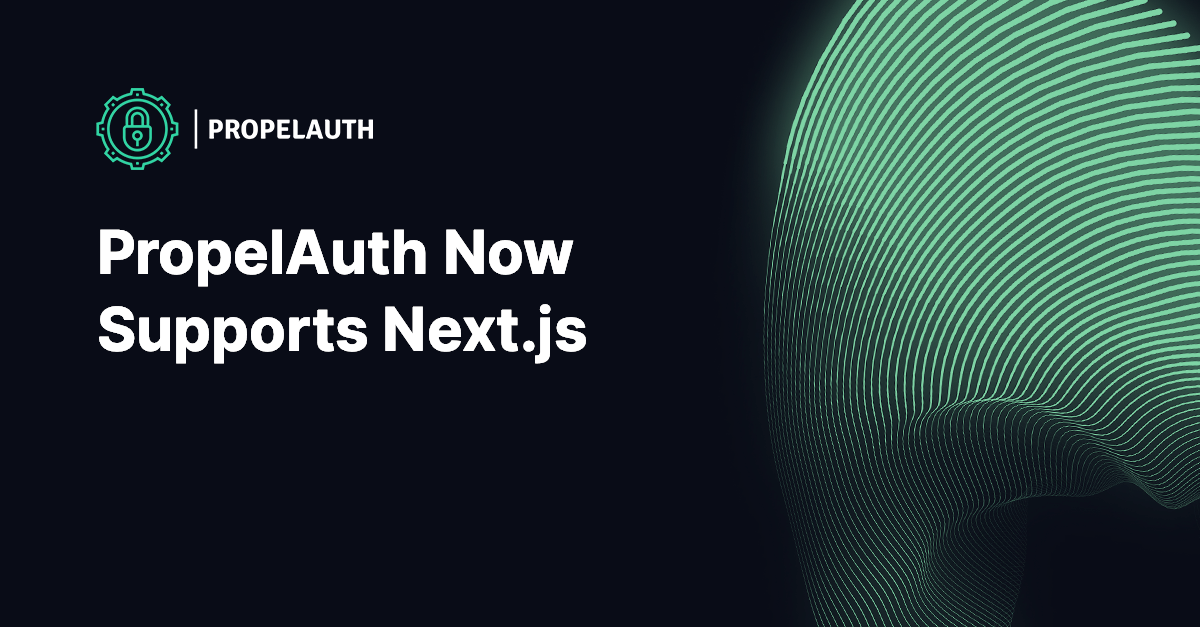
Next.js is a popular React framework. It provides a lot of useful features out of the box like route pre-fetching, built-in CSS support, and a lot more. It also provides API routes which let you build out serverless backend functions.
We're excited to announce that PropelAuth supports Next.js on both the frontend and backend.
With PropelAuth, you can easily build and configure login, signup, account pages, organization management, transactional emails, and more in a matter of minutes. You can then write an API route as simple as:
export default async function handler(req, res) {
// check if the request contains a valid token
await requireUser(req, res)
// req.user is automatically set by requireUser
res.status(200).json({user_id: req.user.userId})
}
to make a protected backend serverless route. You can also write a component as simple as:
function SomeComponent(props) {
// isLoggedIn and user are injected automatically from withAuthInfo below
if (props.isLoggedIn) {
return <div>You are logged in as {props.user.email}</div>
} else {
return <div>You are not logged in</div>
}
}
export default withAuthInfo(SomeComponent);
to access user information on your frontend.
Interested in learning more? Check out our full blog post where we walk you through all the details of building a Next.js app with authentication powered by PropelAuth.